Using Plane.Raycast (Unity)
Optimizing the Raycast to use pure geometry instead of doing Physics Queries to check collisions with colliders.
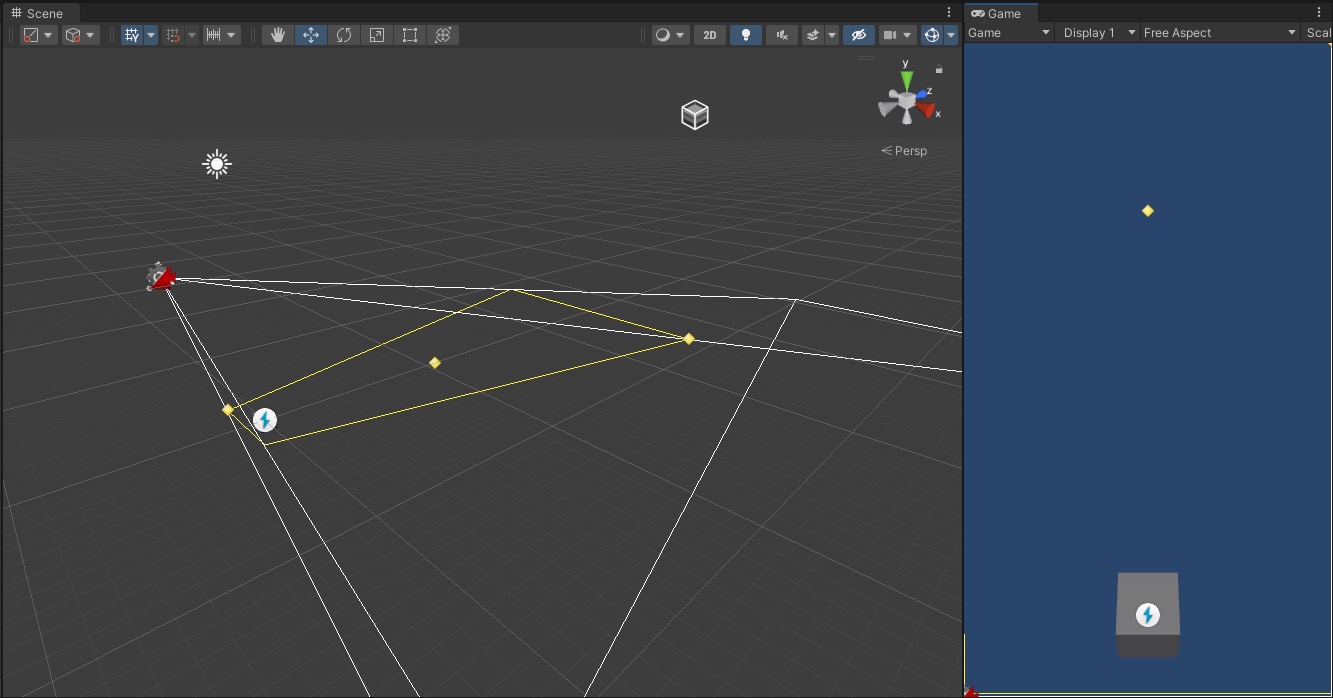
References
Table of Content
Optimization
- This operation is more efficient than the physics based Raycast with colliders.
- Instead of making Physics Queries, and checking for Colliders in the Scene, this operation is purely geometrical.
Raycast to the Plane
- Define a
Plane
using three points. - Use
Camera.ViewportPointToRay
to obtain a ray and raycast it onto the plane. - Obtain the point in space using
ray.getPoint(distance)
.
1public Camera mainCamera; 2public Transform bottomLeft; 3public Transform topRight; 4private Plane plane; 5 6void Awake() 7{ 8 this.plane = new Plane(transform.position, bottomLeft.position, topRight.position); 9 PositionCorner(new Vector2(0.0f, 0.0f), bottomLeft); 10 PositionCorner(new Vector2(1.0f, 1.0f), topRight); 11} 12 13private void PositionCorner(Vector2 viewportPoint, Transform corner) 14{ 15 var distance = 0.0f; 16 var ray = mainCamera.ViewportPointToRay(viewportPoint); 17 if (this.plane.Raycast(ray, out distance)) 18 { 19 corner.transform.position = ray.GetPoint(distance); 20 } 21}
Using the Input System
- Same as before, but using
Camera.ScreenPointToRay
.
1public Camera mainCamera; 2public Plane plane; 3private bool isMoving = false; 4 5public void OnMoveAction(InputAction.CallbackContext context) 6{ 7 isMoving = context.performed; 8} 9 10public void OnPointerPosition(InputAction.CallbackContext context) 11{ 12 if (!isMoving) { return; } 13 14 var point = context.ReadValue<Vector2>(); 15 16 var distance = 0.0f; 17 var ray = mainCamera.ScreenPointToRay(point); 18 if (this.plane.Raycast(ray, out distance)) 19 { 20 transform.position = ray.GetPoint(distance); 21 } 22}